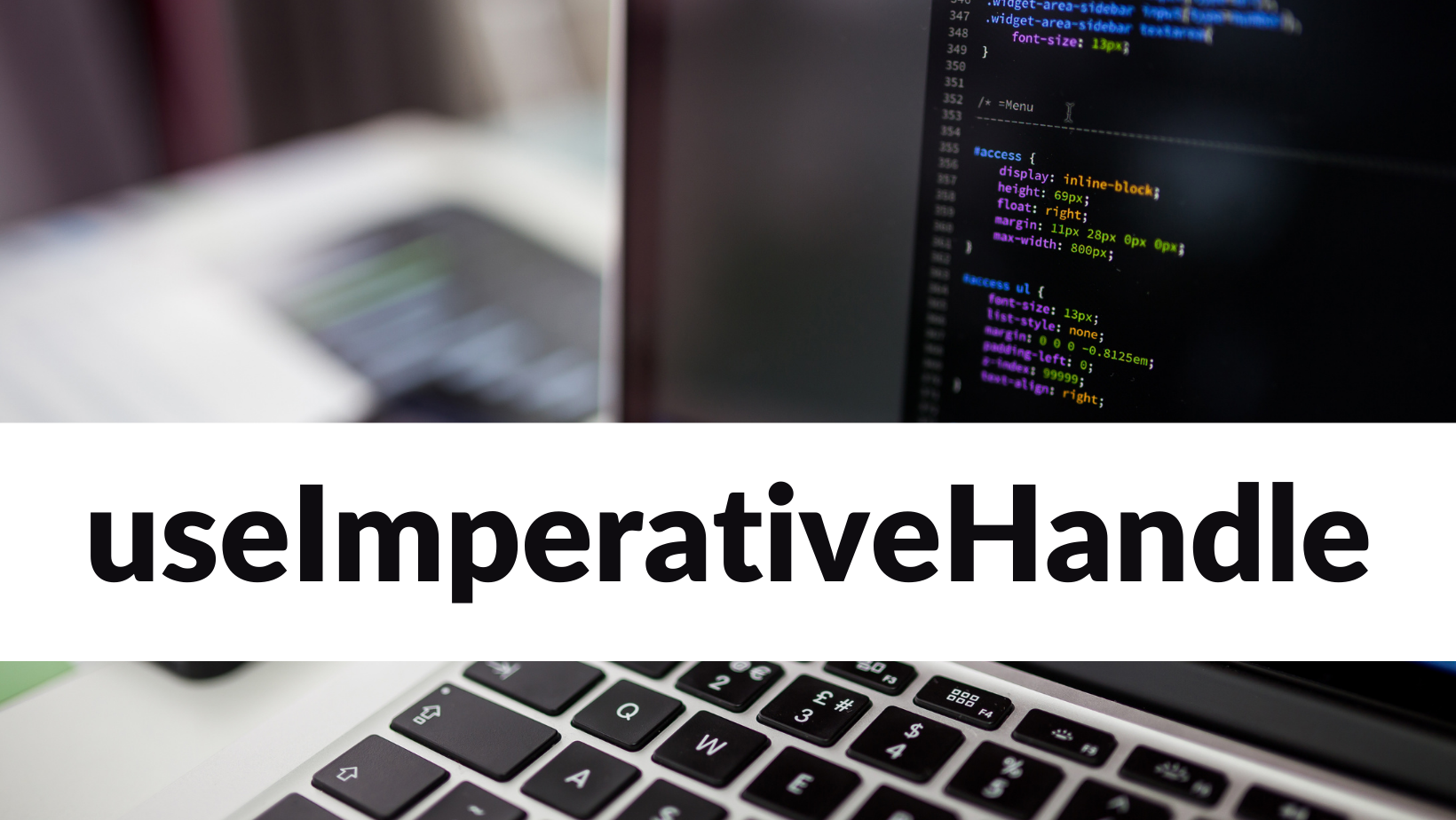
What is useImperativeHandle in React?
02/11/2023
4 min read
In this post, I will introduce you to one of the lesser-known, but very useful React Hooks -
useImperativeHandle
. At first glance, its name may seem a bit daunting, but I guarantee that after reading this post everything will become clear.What is useImperativeHandle?
useImperativeHandle
is a hook that allows a child component to expose certain functions or properties to the parent component, giving it more control over the child component.By default in React, there is only a one-way data flow. This means that props can only be passed from the parent component to the child component. As a result, the parent cannot call methods that are defined in descendant components.
There are situations that require the parent to be able to control elements of the child component, for example:
- Form validation: The child component may expose a function that checks form data to the parent component, which can be used to display error messages or prevent form submission.
- Handling user input data: The child component may expose a function handling user input data to the parent component, which can be used to update other parts of the application state.
- Access to the child state:
useImperativeHandle
can be used to expose certain state values of the child component to the parent component, allowing the parent component to access and use this state.
How is it constructed?
useImperativeHandle
takes two arguments: a reference and a factory function. The factory function returns an object that is available to the parent through ref
.Here is the basic syntax:
useImperativeHandle(ref, () => {// this is where you can define methods}, [deps]);
ref
: Reference passed from the parent component.- A function that exposes the data methods, variables from the component in
ref
. deps
: Dependency array, similar to other hooks.
What is it most useful for? - Real use case from a project
Imagine that in your application you have a video component. It has a thumbnail, player, and a button that allows us to play the video. Such a component can be placed anywhere in the application and it will work.
However, a requirement comes that in one case the video component should become part of another component, e.g. some
Card
component, where there is an additional button that plays the passed video.Acting in the standard way, we would have to move all the video playback logic out of the video component, to all components that use it. We don't want to do this because all the logic behind the video should be in the component.
So how to deal with such a requirement?
In this case,
useImperativeHandle
will work perfectly. In the video component, through this hook, we will expose methods to turn on the video, by which our CardComponent
will have access to them.See the example:
import React, { useRef, useImperativeHandle, forwardRef } from 'react';const VideoComponent = forwardRef((props, ref) => {const [isVideoPlayed, setIsVideoPlayed] = useState(false);useImperativeHandle(ref, () => ({playVideo: () => {setIsVideoPlayed(true);}}));return <VideoPlayer isVideoPlayed={isVideoPlayed} setIsVideoPlayed={setIsVideoPlayed}/>;});const CardComponent = () => {const videoRef = useRef();const onClick = () => {videoRef.current.playVideo();};return (<><VideoComponent ref={videoRef} /><button onClick={onClick}>Play video</button></>);};
In the
VideoComponent
component, we use forwardRef
and useImperativeHandle
to "expose" the playVideo
method to the parent component. Then, in CardComponent
, we can use this method directly.Thanks to this solution, the video playback logic is still encapsulated in the component responsible for it, and we have gained additional possibilities to play the video from outside another component.
However, remember that
useImperativeHandle
should be used sparingly and with caution. Good design principle says that components should be as independent as possible and independent of the parent. Using this hook can lead to too strong dependencies between components. Always think twice before deciding to use it!If you can express something as a prop, you should not use
useImperativeHandle
. For example, instead of exposing such props as { open, close } from the Modal component, it is better to accept isOpen as a prop <Modal isOpen={isOpen} />
and use useEffect
for proper synchronization.